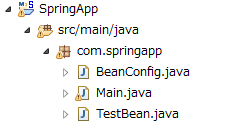
前回は、beanの設定ファイルを用いて、DIを実装してみました。
次は、アノテーションを使って、設定用のクラスを作りそこから注入するサンプルをご紹介します。
最近のSpringによるDIは、Bean設定ファイルによるものよりも、アノテーションによる設定クラスを作って注入する形が主となってきています。是非、こちらも覚えておきましょう。
サンプル
アノテーションによる設定用クラス
1 2 3 4 5 6 7 8 9 10 11 12 |
import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; @Configuration public class BeanConfig { @Bean public TestBean configBean(){ return new TestBean("Hello World!"); } } |
アノテーションの説明
アノテーション名 | 説明 |
---|---|
@Configuration | Beanの設定を行うクラスであることを示します。(クラスの先頭につける。) |
@Bean | これをつけたメソッドは、Beanを作成するものと認識される。戻り値として必ずBeanクラス名にする。(メソッドの先頭につける。) |
設定を注入するBeanクラス
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
public class TestBean{ private String helloworld; public TestBean(String helloworld){ this.helloworld = helloworld; } public String getHelloworld() { return helloworld; } public void setHelloworld(String helloworld) { this.helloworld = helloworld; } } |
実行用クラス
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
import org.springframework.context.ApplicationContext; import org.springframework.context.annotation.AnnotationConfigApplicationContext; public class Main { public static void main(String[] args) { ApplicationContext app = new AnnotationConfigApplicationContext(BeanConfig.class); TestBean beantest = (TestBean)app.getBean(TestBean.class); System.out.println(beantest.getHelloworld()); } } |
「AnnotationConfigApplicationContext(設定ファイルのクラス.class);」と指定することで、注入する設定インスタンスを取得することができます。
プログラム構成
設定ファイルがなくなりましたね。
実行結果
コンポーネントを使う。
コンポーネント用クラス
1 2 3 4 5 6 7 8 9 10 11 12 |
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Component; @Component public class BeanComponent { @Autowired private TestBean bean; public void getHello() { System.out.println(bean.getHelloworld()); } } |
使っているアノテーション
アノテーション名 | 説明 |
---|---|
@Component | そのクラスがコンポーネントであることを指す。 |
@Autowired | コンフィグクラスから、インスタンスを探して自動的に代入してくれる。 |
コンフィグクラス
基本は、上でご紹介したクラスと同じですが、「@ComponentScan」アノテーションをクラスの前に追加しています。
これをつけることで、コンポーネントを検索して、インスタンス化してApplicationContextに登録してくれる。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.ComponentScan; import org.springframework.context.annotation.Configuration; @Configuration @ComponentScan public class BeanConfig { @Bean public TestBean configBean(){ return new TestBean("Hello World!"); } } |
実行用クラス
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
import org.springframework.context.ApplicationContext; import org.springframework.context.annotation.AnnotationConfigApplicationContext; public class Main { public static void main(String[] args) { ApplicationContext app = new AnnotationConfigApplicationContext(BeanConfig.class); BeanComponent comp = app.getBean(BeanComponent.class); comp.getHello(); } } |
この記事へのコメントはありません。